ES2015: Promise, Async/Await
Date: 22 Feb 2023
• Promises are an alternative to callbacks for delivering the results of an asynchronous computation.
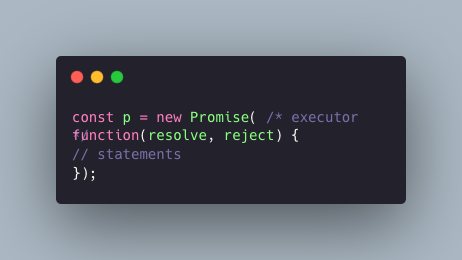
• A Promise is always in one of three mutually exclusive states: - Before the result is ready, the Promise is pending. - If a result is available, the Promise is fulfilled. - If an error happened, the Promise is rejected.
• A Promise is settled if “things are done” (if it is either fulfilled or rejected).
• A Promise is settled exactly once and then remains unchanged.
• Promise reactions are callbacks that you register with the Promise method then(), to be notified of fulfillment or a rejection.
• A thenable is an object that has a Promise-style then() method. Whenever the API is only interested in being notified of settlements, it only demands thenables (e.g. the values returned from then() and catch(); or the values handed to Promise.all() , Promise.allSettled(), Promise.race()), Promise.race()).
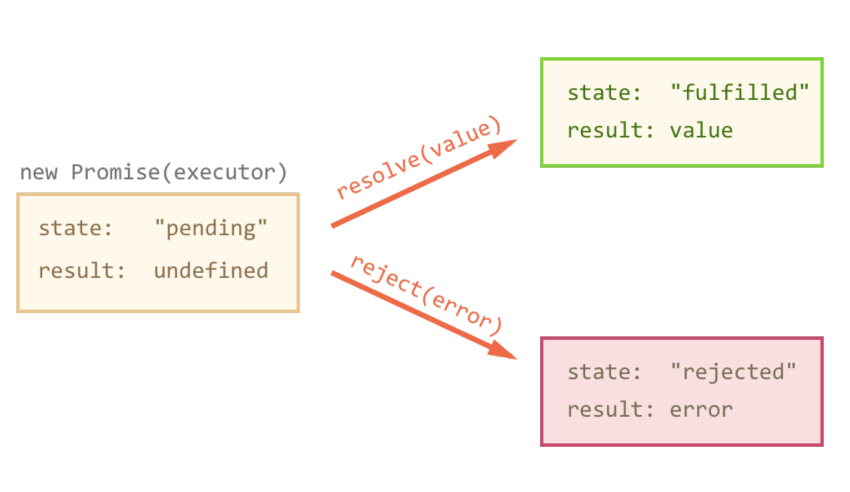
• Create a promise
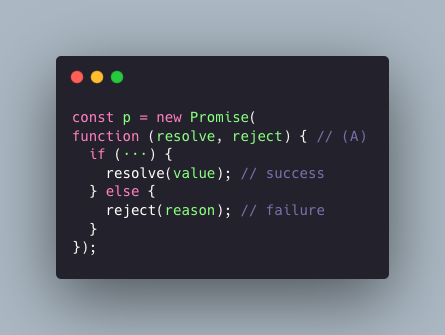
• Consuming a Promise
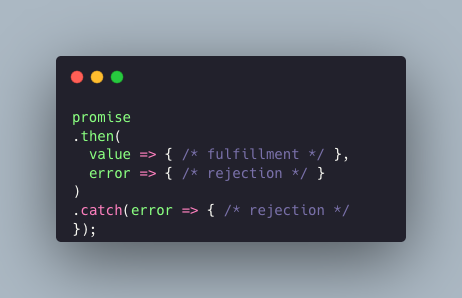
• Other ways of creating Promises - Promise.resolve() - Promise.reject()
• Chaining Promises
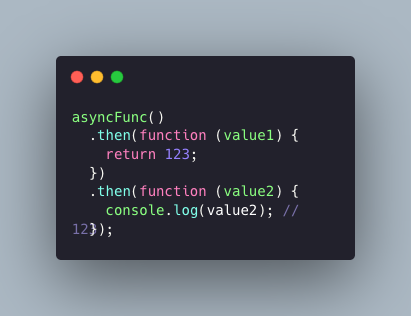
• Promise anti-pattern: nested
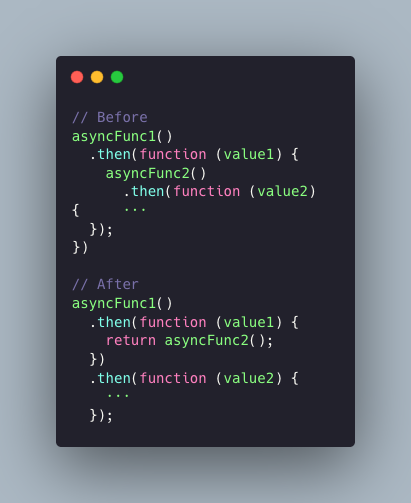
• then always return a promise
• Async functions: always return a promise
• Await: works only inside async functions
• Can’t use await in regular functions
• await won’t work in the top-level code
• Error handling
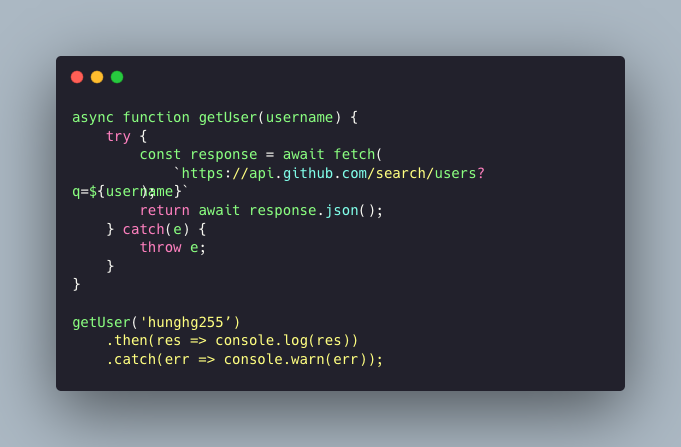
• Too Sequential
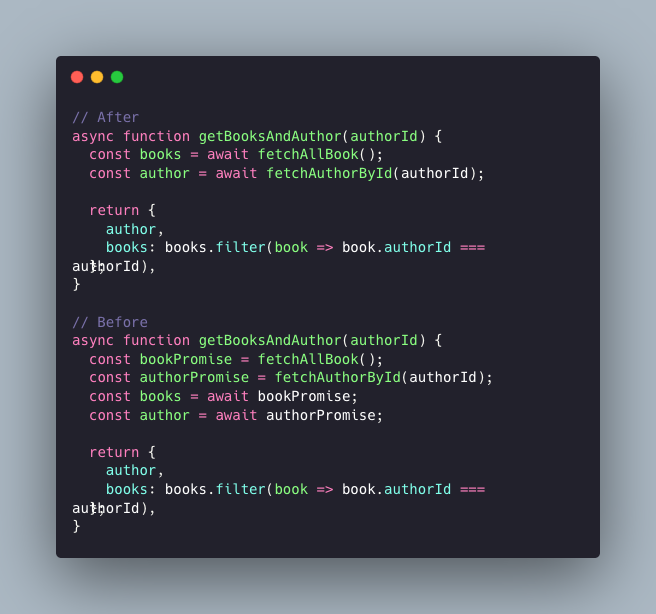
• http://speakingjs.com/es5/index.html
• http://exploringjs.com/es6/index.html
• http://exploringjs.com/es2016-es2017/ch_async-functions.html
• http://exploringjs.com/es2018-es2019/toc.html
• http://eloquentjavascript.net/
• https://github.com/getify/You-Dont-Know-JS#titles