Data type, Operators, Variables, Scope, Program Structure
Date: 15 Feb 2023
1. Primitive Values.
• Boolean: true/false
• Number: 10, 3.14, ...
• String: ‘abc’, ...
• Null
• Undefined
• Symbol (ES2015)
Note: You can’t define your own primitive types.
Compared by value: The content is compared
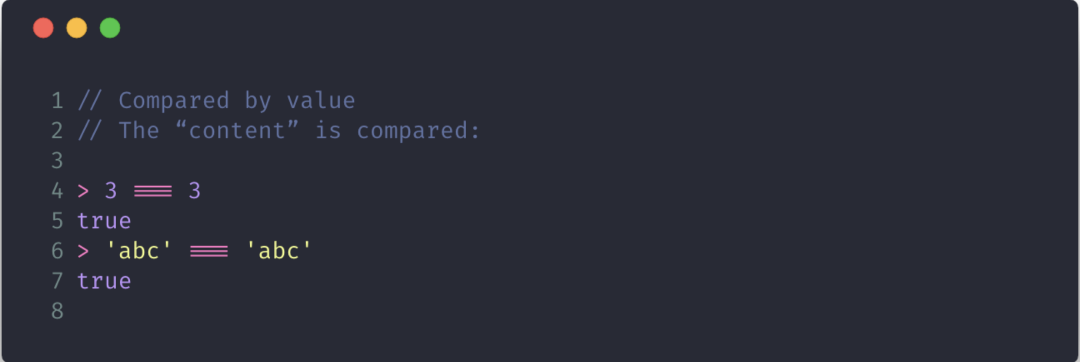
Always immutable
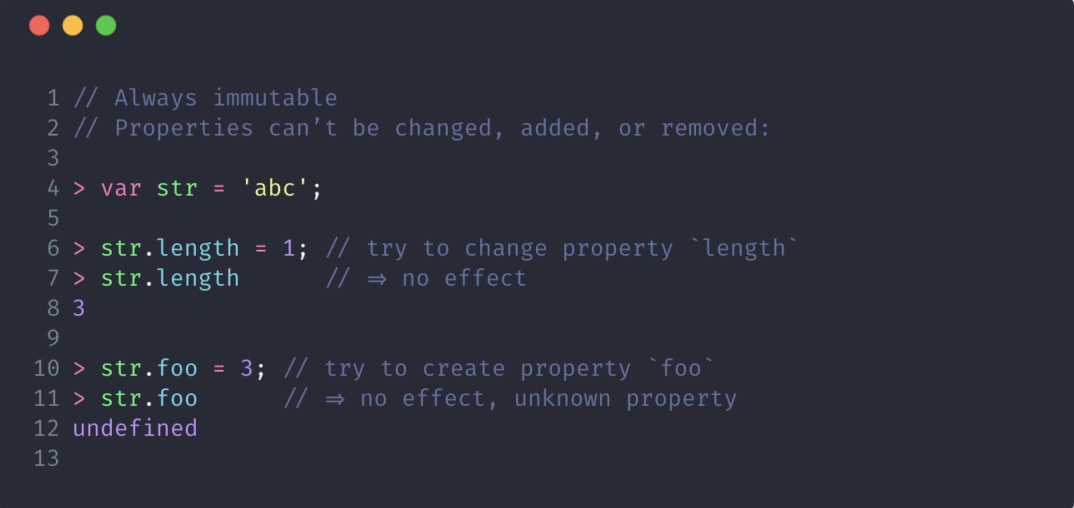
2. Reference Values
All nonprimitive values are objects.
Compared by reference
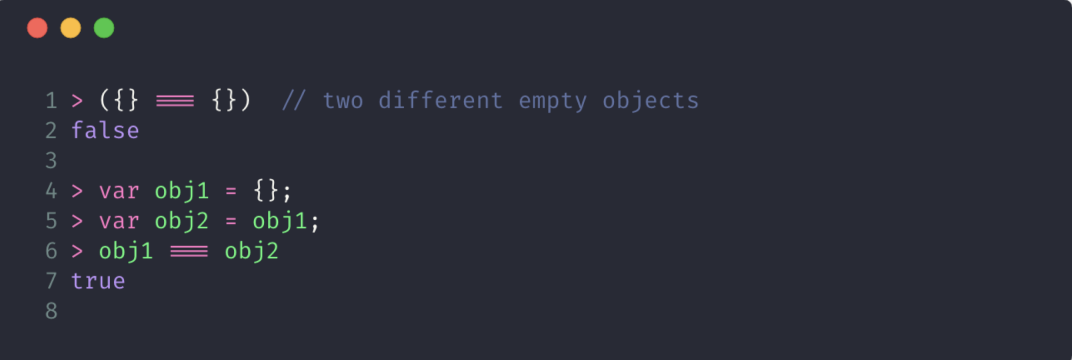
Mutable by default
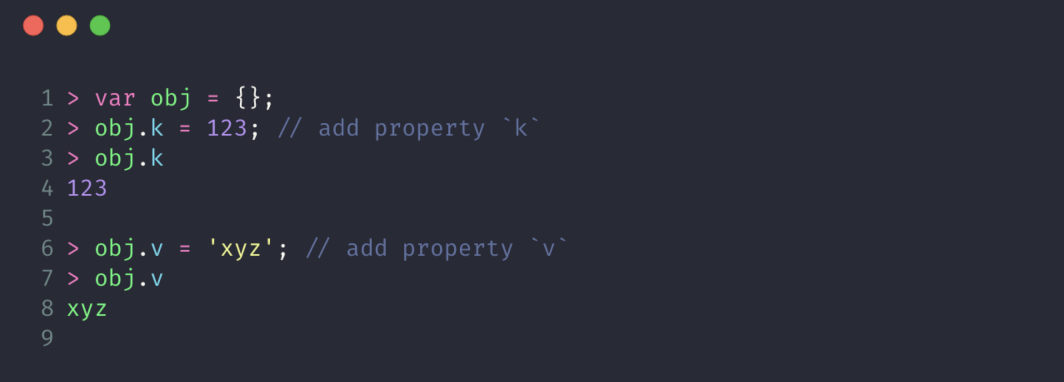
User-extensible
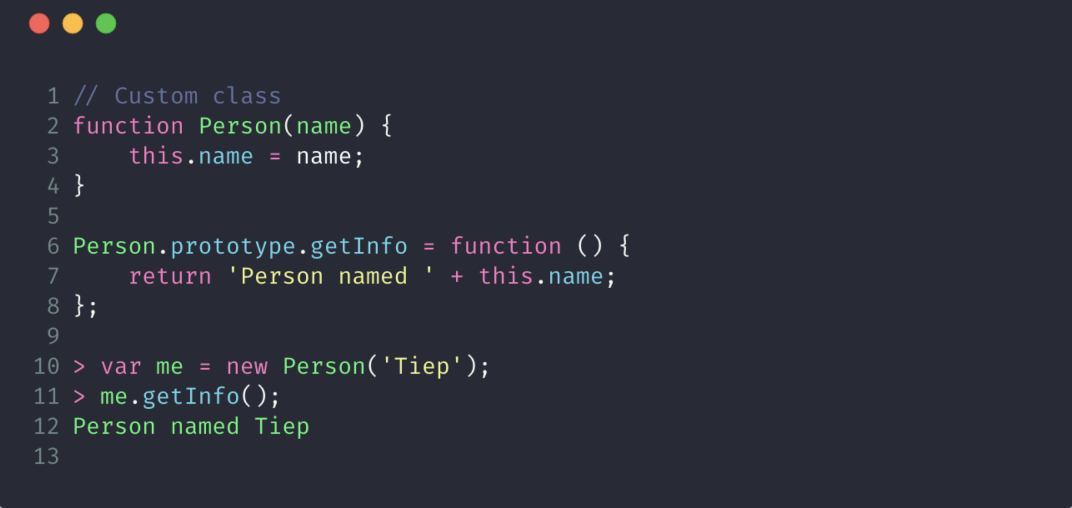
3. Null and Undefined
• undefined means “no value” (neither primitive nor object). - Uninitialized variables - Missing parameters - Missing properties have a nonvalue - Functions implicitly return it if nothing has been explicitly returned. • null means “no object.” It is used as a nonvalue where an object is expected (as a parameter, as a member in a chain of objects, etc.).
• undefined and null are the only values for which any kind of property access results in an exception.
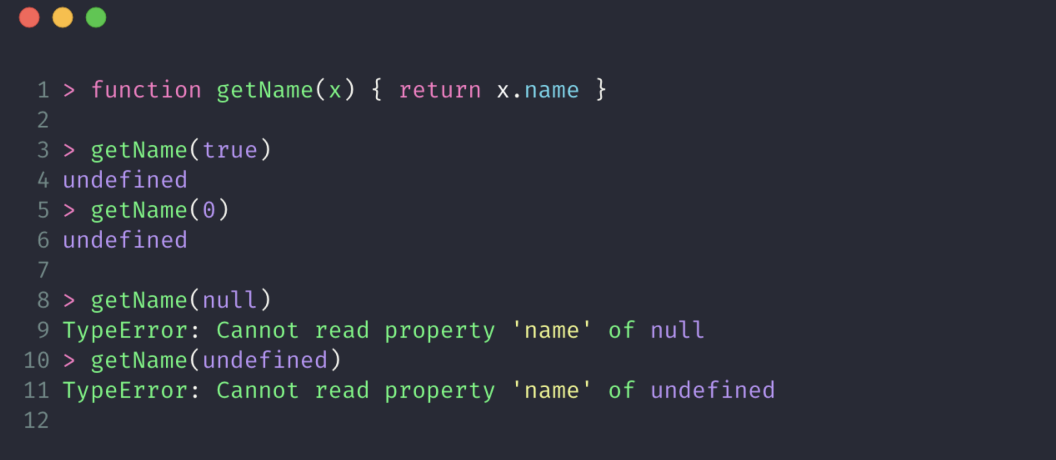
• undefined is not a keyword in JavaScript.
4. Truthy and Falsy Values
• undefined, null
• Boolean: false
• Number: 0, NaN
• String: ''
• all objects are truthy
5. Operators
• Assignment operators
• Comparison operators
• Arithmetic operators
• Bitwise operators
• Logical operators
• String operators
• Conditional (ternary) operator
• Comma operator
• Unary operators
• Relational operators
• JavaScript Comparison Algorithms:
- Two strings are strictly equal when they have the same sequence of characters, same length, and same characters in corresponding positions. - Two numbers are strictly equal when they are numerically equal (have the same number value). NaN is not equal to anything, including NaN. Positive and negative zeros are equal to one another.
- Two Boolean operands are strictly equal if both are true or both are false.
- Two distinct objects are never equal for either strict or abstract comparisons. - An expression comparing Objects is only true if the operands reference the same Object. - Null and Undefined Types are strictly equal to themselves and abstractly equal to each other.
• JavaScript Comparison Algorithms - Equality Operators:
- When comparing a number and a string, the string is converted to a number value.
- If one of the operands is Boolean, (1 if it is true and +0 if it is false). - If an object is compared with a number or string, JavaScript attempts to return the default value for the object. Operators attempt to convert the object to a primitive value, a String or Number value, using the valueOf and toString methods of the objects. If this attempt to convert the object fails, a runtime error is generated.
- If the valueOf method returns a primitive value, the toString method will never be called.
- Note that an object is converted into a primitive if, and only if, its comparand is a primitive. If both operands are objects, they're compared as objects, and the equality test is true only if both refer to the same object.
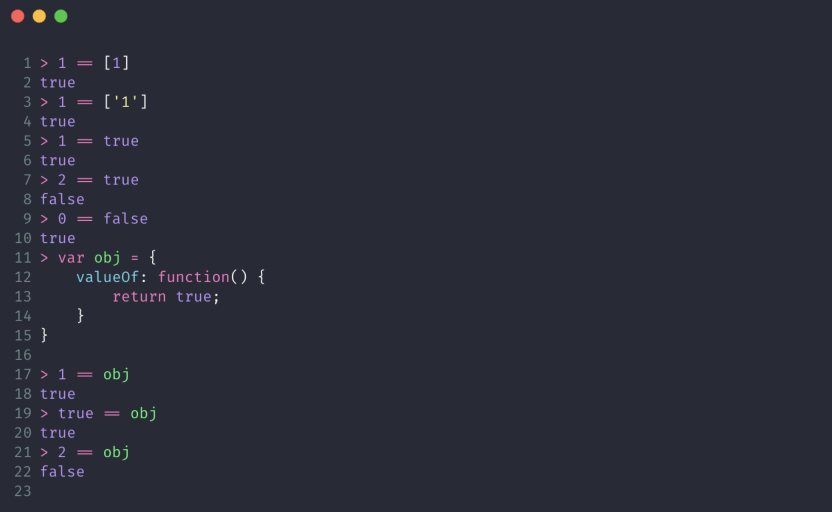
6. The Plus Operator
• If one of them is a string, the other is also converted to a string and both are concatenated.
• Otherwise, both operands are converted to numbers and added.
7. Unary Operators
• Unary plus (+): Tries to convert the operand into a number • Unary negation (-): Tries to convert the operand into a number and negates after • Logical Not (?): Converts to boolean value then negates it • Increment (++): Adds one to its operand • Decrement (--): Decrements by one from its operand • Bitwise not (~): Inverts all the bits in the operand and returns a number • typeof: Returns a string which is the type of the operand • delete: Deletes specific index of an array or specific property of an object • void: Discards a return value of an expression.
8. Variables, Scope
• Declaring and Assigning Variables.
• Function scope: var
• Block scope: let, const
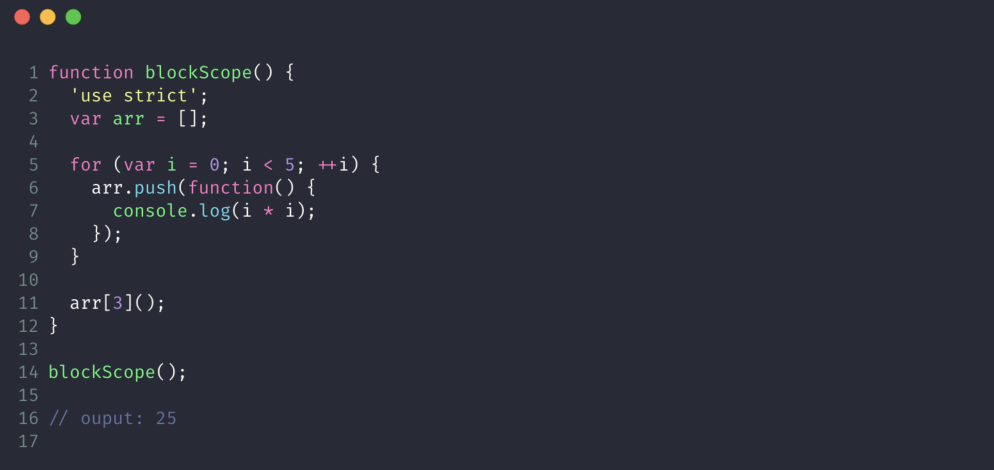
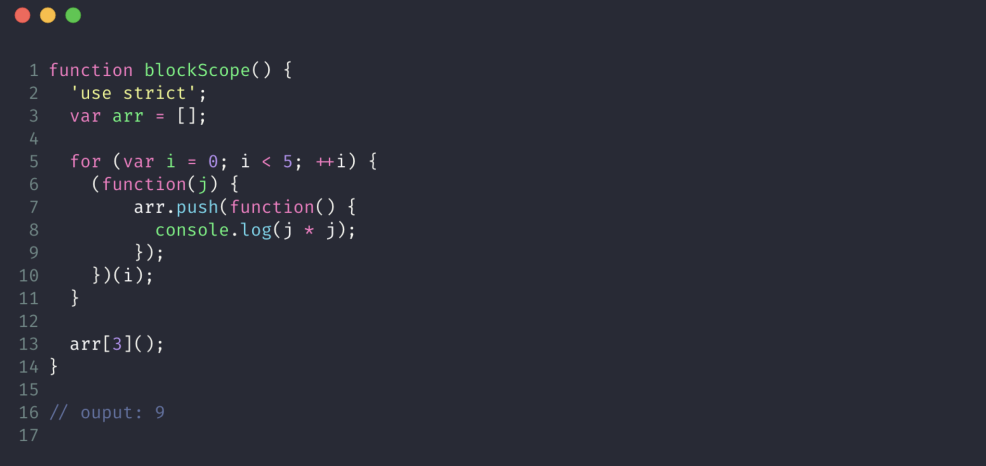
9. Program Structure
• Conditionals: if-else, switch-case.
• Loops: while, do-while, for, for-in, for-of.
• http://speakingjs.com/es5/index.html
• http://exploringjs.com/es6/index.html
• http://exploringjs.com/es2016-es2017/ch_async-functions.html
• http://exploringjs.com/es2018-es2019/toc.html
• http://eloquentjavascript.net/
• https://github.com/getify/You-Dont-Know-JS#titles