Asynchronous Programming
Date: 22 Feb 2023
1. Blocking and non-blocking
• Blocking: like some c/c++ languages... the code is executed from top to bottom from left to right. Some works will be waiting for a long time such as reading, and writing files, ...
• Non-blocking: in javascript code will still run from top to bottom from left to right but there will be some tasks such as timeout, ajax, and DOM,..., these tasks in javascript will not execute directly. it will be passed to the web API to process and return a callback function, the callback functions will be executed later
2. Asynchronous Programming
• Both JavaScript in the browser, and in Node.js, are single-threaded.
• In synchronous programs, if you have two lines of code (L1 followed by L2), then L2 cannot begin running until L1 has finished executing.
• In asynchronous programs, you can have two lines of code (L1 followed by L2), where L1 schedules some tasks to be run in the future, but L2 runs before that task completes.
• Call Stack
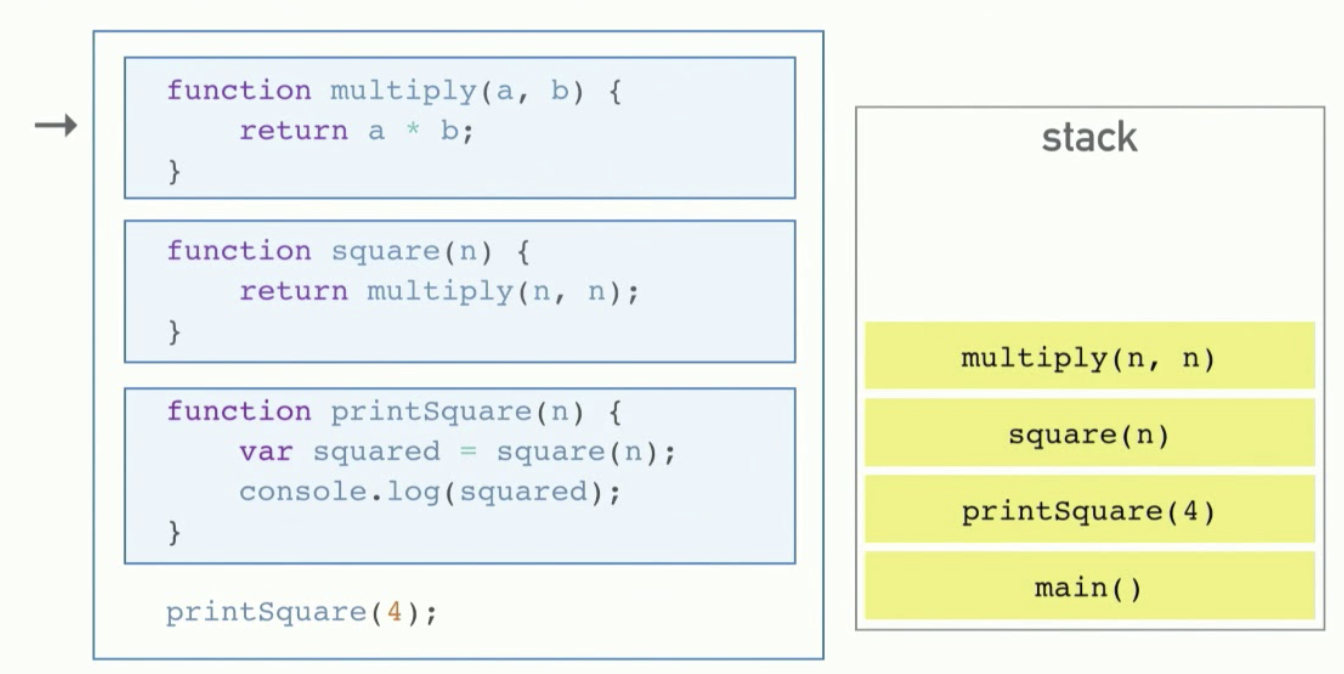
• Web Browser API
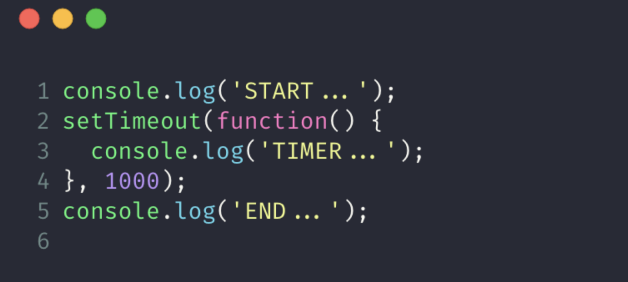
• Event Loop: The reality is that all JavaScript executes synchronously - it's the event loop that allows you to queue up an action that won't take place until the loop is available some time after the code that queued the action has finished executing.
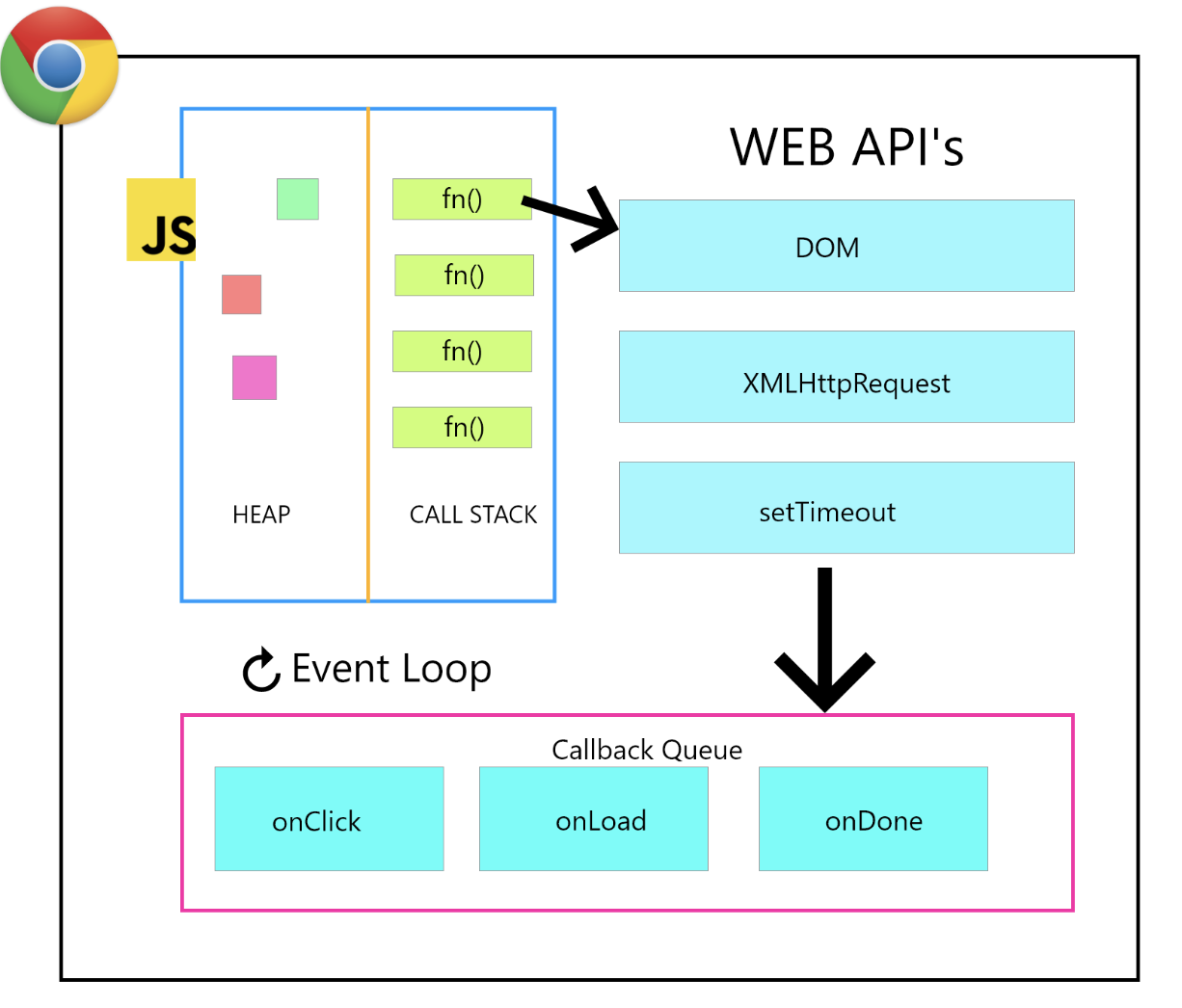
• Callback:
- Pros: Easy to understand (only for simple callback function). - Cons: Error handling becomes more complicated, Composition is more complicated (Callback hell)
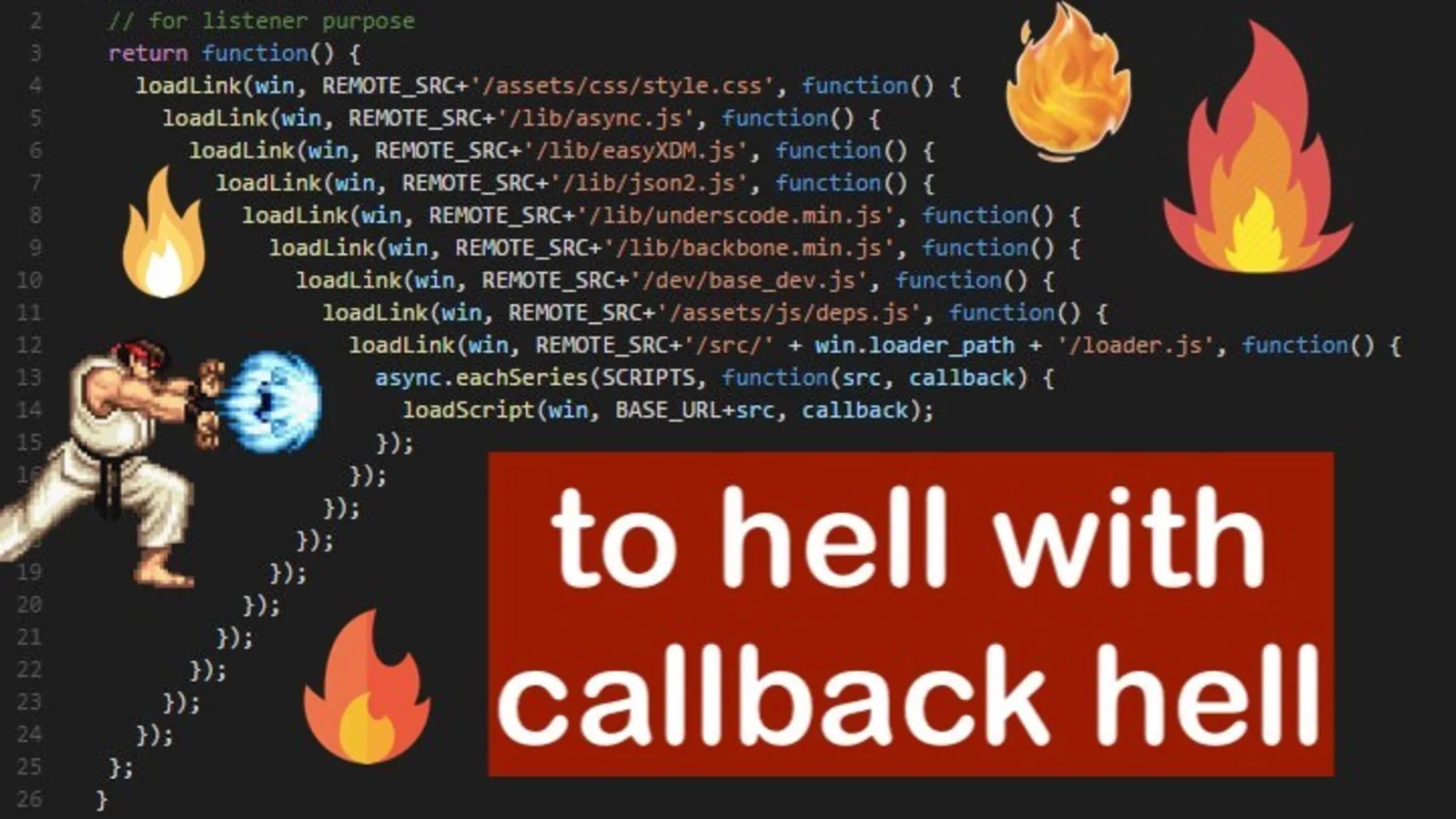
• AJAX
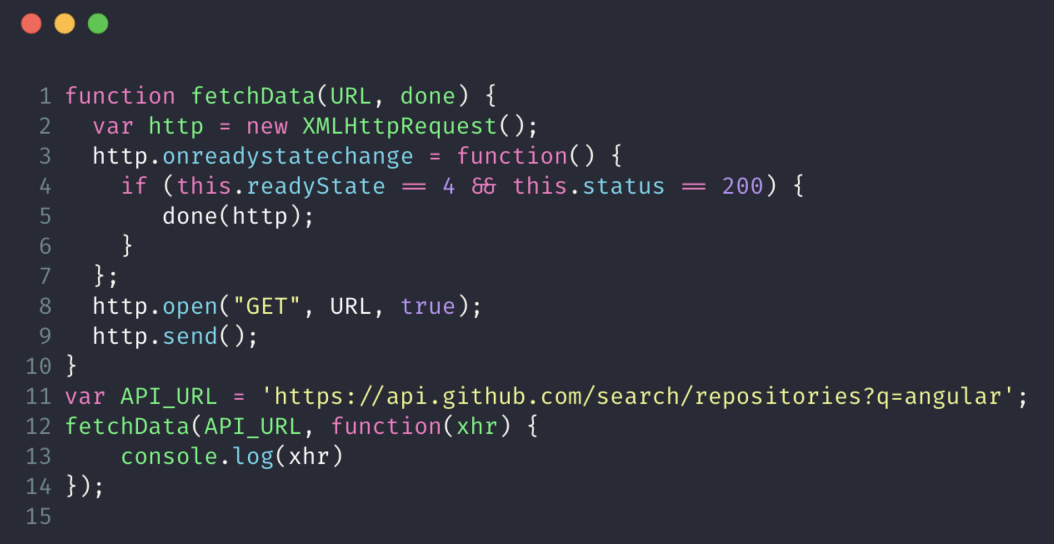
• Asynchronous programming makes it possible to express waiting for long-running actions without freezing the program during these actions.
• JavaScript environments typically implement this style of programming using callbacks, functions that are called when the actions complete. • An event loop schedules such callbacks to be called when appropriate, one after the other, so that their execution does not overlap.
• http://speakingjs.com/es5/index.html
• http://exploringjs.com/es6/index.html
• http://exploringjs.com/es2016-es2017/ch_async-functions.html
• http://exploringjs.com/es2018-es2019/toc.html
• http://eloquentjavascript.net/
• https://github.com/getify/You-Dont-Know-JS#titles